- Out-of-Stock
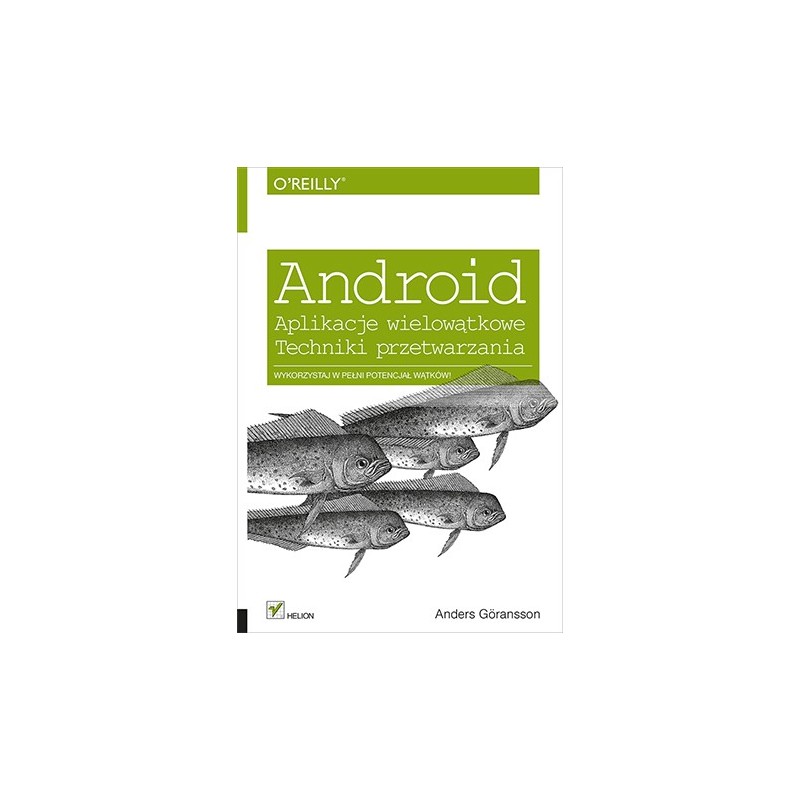
Anders Göransson
free shipping in Poland for all orders over 500 PLN
If your payment will be credited to our account by 11:00
Each consumer can return the purchased goods within 14 days
Original title: Efficient Android Threading: Asynchronous processing techniques for Android applications
Translation: Lech Lachowski
ISBN: 978-83-246-9614-7
Date of issue: 2015-02-24
Format: 168x237
Pages: 248
Make the most of the threads!
If you want to create applications that provide users with great experience, if you want to do complex tasks faster or you just have to do different things at the same time - learn how to use threads. Creating multithreaded programs is difficult, but mastering this art will allow you to achieve tangible benefits!
This book is entirely devoted to the use of threads on the Android platform. Thanks to it you will get to know various methods of asynchronous processing and their advantages and disadvantages. However, at the very beginning you will get acquainted with the basic information on Java multithreading. You will learn how threads communicate with each other and synchronize access to resources and how to manage them. The following chapters contain a large dose of knowledge about various asynchronous techniques. Familiarization with their content will help you choose a technique that will meet your requirements when you start developing a multi-threaded application. This book is a must-read for programmers who want to take full advantage of the Android platform.
Guide to the world of threads on the Android platform!
Table of Contents
Foreword (11) 1. Components of the Android system and the need for multiprocessor processing (15)
PART I. BASIS (27) 2. Multithreading in Java (29)
3. Threads in the Android system (41)
4. Thread communication (49)
5. Communication between processes (81)
6. Memory management (93)
PART II. ASYNCHRONIC TECHNIQUES (107) 7. Basic thread life cycle management (109)
8. HandlerThread class: high-level queuing mechanism (121)
9. Checking the execution of a thread using the artist's framework (131)
10. Binding a background task to a user interface thread using the AsyncTask class (151)
11. Services (169)
12. IntentService class (189)
13. Accessing the ContentProvider class using the AsyncQueryHandler class (197)
14. Automatic background execution using chargers (207)
15. Summary: selection of asynchronous technique (229)
A. Bibliography (235) Index of reference (237)
No product available!
No product available!
No product available!
No product available!
Female terminal block, 4-pin. Pitch 3.81mm. Height 11.1mm. 15EDGK-3.81-4P
No product available!
No product available!
No product available!
No product available!
HK 10x4.7 SF Carbon Fiber Propellers L/H and R/H Rotation (1 pair) (39773)
No product available!
Pololu m3pi Robot with mbed SocketPowerful Polol 3pi robot work. Line Follower mobile platform with two motors, 5 reflection sensors, LCD display, buzzer and three user buttons as well as mbed extension board. Pololu 2151
No product available!
No product available!
No product available!
No product available!
AUTOMOBILE ALARM CONTROL PANEL WITH VARIAGE PASSWORD AND RS485 - PLATEBOARD AND PROGRAMMED LAYOUT
No product available!
Miniature, remote controlled robot (Mini R / C Battle Robot) with RC 2.4GHz remote control, charger and traffic lights. Black colour.
No product available!
No product available!
Anders Göransson