- Out-of-Stock
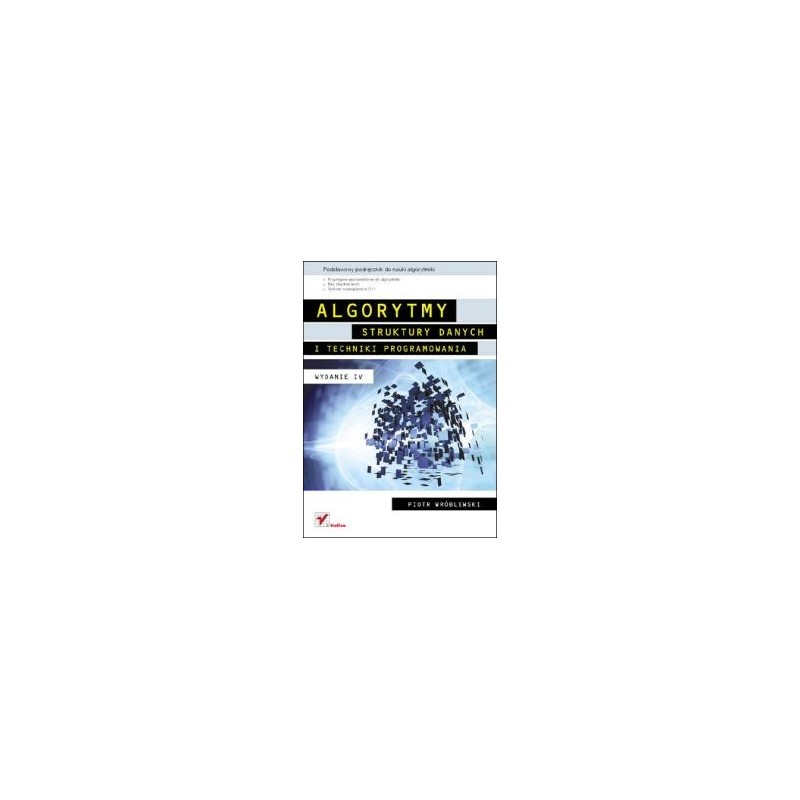
free shipping in Poland for all orders over 500 PLN
If your payment will be credited to our account by 11:00
Each consumer can return the purchased goods within 14 days
No product available!
No product available!
No product available!
No product available!
No product available!
No product available!
Male terminal block, angled, 10-pin. Pitch 3.81mm. Height 7mm. 15EDGRC
No product available!
No product available!
No product available!
Assembled adjustable power supply for contact plates. AVT1990 C
No product available!
A module for building electronic organs in the form of a kit for self-assembly. The board is based on the NE555 system, it is supplied with the voltage from 3 to 12 V
No product available!
No product available!
No product available!
A assembled LED bauble for everyone. AVT3250 C
No product available!
No product available!
This synchronous switching step-down (or buck) regulator takes an input voltage of up to 38 V and efficiently reduces it to 5 V with an available output current of around 6 A.
No product available!