- Out-of-Stock
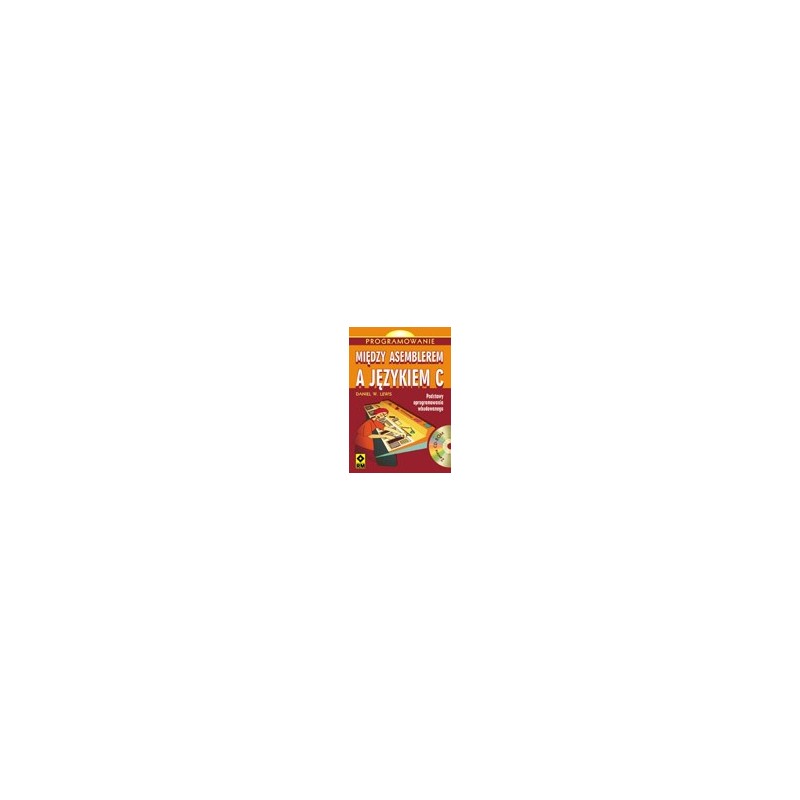
free shipping in Poland for all orders over 500 PLN
If your payment will be credited to our account by 11:00
Each consumer can return the purchased goods within 14 days
Author: Daniel W. Lewis ISBN: 83-7243-412-3 Format: B5, 300 pages Includes CD-ROM Publisher: RM Original edition: Fundamentals of Embedded Software: Where C and Assembly Meet Prentice Hall |
About the book |
This book is a great alternative to traditional university texts on computer organization and programming in assembler language. Assembler appears in it as it is most often used in practice - as a tool to implement small, fast and specialized procedures called from a main program written in a high level language, such as C. Based on embedded software, the book introduces multithreaded programming techniques, systems with expropriation and expropriation, shared resources and scheduling, thus providing a solid foundation for further learning of operating systems, real-time systems and microprocessor-based design. The book should help:
Table of Contents Preface Chapter 1 Introduction 1.1 What is a built-in system? Chapter 2 Data representation 2.1 Binary numbers with constant precision Chapter 3 Maximum use of C 3.1. Total types Chapter 4 The programmer's view on building a computer 4.1 Memory Chapter 5 Combining C language and assembly language 5.1 Programming in assembler Chapter 6 Programming the input / output 6.1 I / O instructions for Intel processors Chapter 7 Concurrent software 7.1 Multi-level systems Chapter 8 Sorting tasks 8.1 Thread states Chapter 9 Memory management 9.1 Objects in C language Chapter 10 Shared memory 10.1 Identifying shared objects Chapter 11 System initialization 11.1. Organization of memory Appendix A Contents of the CD-ROM Appendix B Compiler C / C ++ DJGPP Installation Appendix C NASM Assembler Installation Appendix D Program designs CD files required by all applications Appendix E libepc library Memory organization and initialization Appendix F Pre-loading program Index |
HK HobbyKing Brushless Car ESC 45A w/ Reverse (13445)
No product available!
No product available!
ROSA3D filament made of high-quality PET-G granules. 3 kg of filament with a diameter of 1.75 mm is wound on the spool. ROSA3D PET-G Standard Black
No product available!
No product available!
Printed circuit board and programmed circuit for a remote radio switch. AVT5590 A +
No product available!
Genesys Virtex-5 FPGA Development Board - development kit
No product available!
No product available!
No product available!
TRANSOFON - A SYSTEM TO CHANGE THE HEIGHT OF A SOUND - A KIT FOR INDEPENDENT INSTALLATION
No product available!
Dispensing needle for precise application of glue, flux with an internal diameter of 1.5mm and an external diameter of 1.8mm.
No product available!
No product available!
AVT kit for self-assembly of the (half) home automation system - four-channel ON / OFF module. AVT3212 B
No product available!
16-button 4 × 4 matrix keyboard module
No product available!
No product available!
No product available!
No product available!