- Out-of-Stock
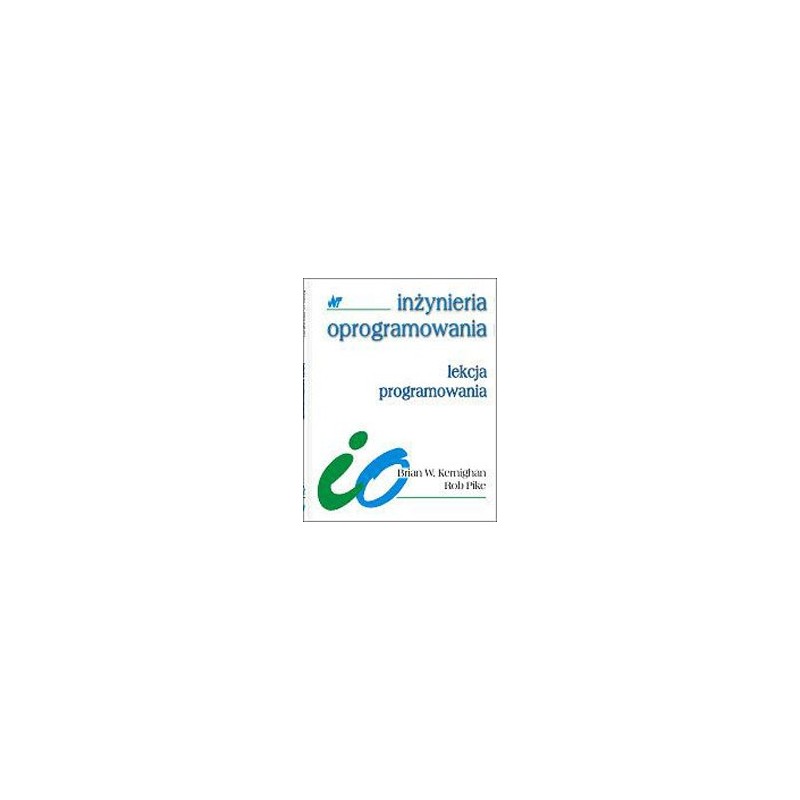
free shipping in Poland for all orders over 500 PLN
If your payment will be credited to our account by 11:00
Each consumer can return the purchased goods within 14 days
Authors: Brian W. Kernighan, Rob Pike ISBN: 83-204-2732-0 Format: 185x235, 318 pages Hardcover Publisher: WNT |
About the book |
Here is a book that should become a compulsory reading of every student learning to program and every professional programmer. The authors - the great global authorities in the field of programming - present the basic principles to be followed if you want to write good programs. They talk about quick and methodical error finding, about proper testing of programs, about taking care of their effectiveness, ensuring their transferability, their proper design, methods of developing interfaces, programming style and taking appropriate notation. They try to make the reader aware that programming is more than writing code. Here are their words: The task of the programmer - regardless of the language used - is to do the best work with the tools that he has at hand. A good programmer can handle a poor language or a rogue operating system, but even the best programming environment will not save a weak programmer Table of Contents Preface Chapter 1: Style 1.1. Names 1.2 Expressions and instructions 1.3. Uniform style and idioms 1.4. Macro instructions as a function 1.5 Magic numbers 1.6 Comments 1.7. Why care about the style Chapter 2. Algorithms and data structures 2.1. Search 2.2 Sorting 2.3 Libraries 2.4. Quick sorting in Java 2.5. Notation 2.6 Expansible tables 2.7 Letters 2.8 Trees 2.9 Distributed tables 2.10 Summary Chapter 3. Design and implementation 3.1 Markov chain algorithm 3.2. Selecting the data structure 3.3. Creating a data structure in C 3.4. Generating the output text 3.5 Java 3.6. C ++ language 3.7 Awk and Perl 3.8 Performance 3.9 Applications Chapter 4. Interfaces 4.1. CSV format - values separated by commas 4.2. Library prototype 4.3 Library for others 4.4. Implementation in C ++ 4.5. Principles of interface design 4.6 Resource management 4.7 Procedure in the event of an error 4.8 User interfaces Chapter 5. Error detection 5.1. Startup programs 5.2 Good tracks, easy mistakes 5.3 No tracks, difficult mistakes 5.4 When everything else has failed 5.5. Unique errors 5.6. Startup tools 5.7 Someone's mistakes 5.8 Summary Chapter 6. Testing 6.1 Test the program as you type 6.2 Systematic testing 6.3 Automated testing 6.4 Test platforms 6.5 Pressure tests 6.6 Some good advice 6.7 Who is testing 6.8 Testing the markov program 6.9 Summary Chapter 7. Performance 7.1 Bottleneck 7.2 Measurement of execution time and program profiling 7.3 Acceleration strategies 7.4 Tuning the code 7.5 Saving memory space 7.6. Estimation 7.7 Summary Chapter 8. Portability 8.1 Language 8.2. Header files and libraries 8.3. Organization of the program 8.4 Isolation 8.5 Data exchange 8.6 Bytes sequence 8.7 Portability and updating 8.8. The international aspect 8.9 Summary Chapter 9. Notation 9.1 Data formatted 9.2 Regular expressions 9.3 Programmable tools 9.4 Interpreters, compilers and virtual machines 9.5 Programs that write programs 9.6 Using macroinstruction to create code 9.7 Compiling in passage Epilogue Appendix: Collected rules Index |
Shielded FTP category 5E Ethernet network cable with RJ45 plugs. Lanberg PCF5-10CC-0025-Y
No product available!
No product available!
Dispensing needle for precise application of glue, flux with an internal diameter of 1.37mm and an external diameter of 1.8mm.
No product available!
No product available!
No product available!
No product available!
No product available!
No product available!
No product available!
No product available!
No product available!
The DC motor driver module makes it possible to control the speed and the direction of rotation using the PWM signal, it has a built-in DRV8835 system. MOD-57
No product available!
No product available!
The clamp meter is equipped with a power and harmonic measurement function. It can measure voltage, current, reactive power, power factor and phase angle. Uni-T UT243
No product available!
No product available!
No product available!