- Out-of-Stock
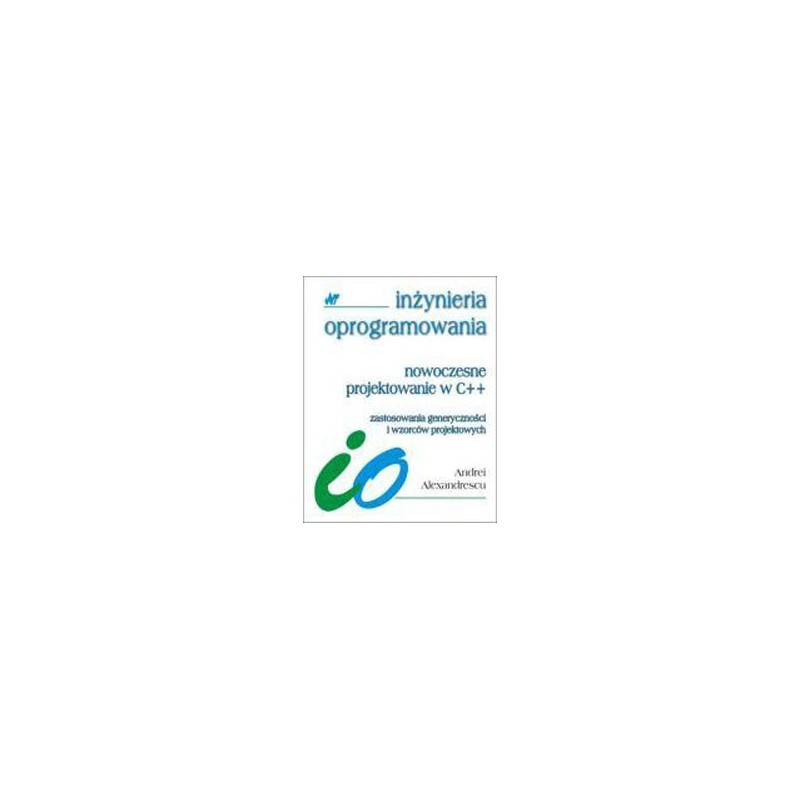
free shipping in Poland for all orders over 500 PLN
If your payment will be credited to our account by 11:00
Each consumer can return the purchased goods within 14 days
Author: Andrei Alexandrescu ISBN: 83-204-3052-6 Format: B5, 362 pages Publisher: WNT |
About the book |
The book is devoted to design patterns, generic programming and C ++ programming language. The right combination of these three elements makes it easy for developers to create a compact and flexible code that can be reused. The author introduces the concept of genetic components - design templates intended for generating repetitive pieces of code. They enable a simple and smooth transition from design to implementation and creating a code that better reflects the original design idea. They facilitate the re-use of design structures, often without the need for any changes. The author describes selected mechanisms and properties of the C ++ language used to create generic components. Analyzes problems that appear in the daily work of a programmer. It presents an innovative technique of class design, namely designing on the basis of guidelines, as well as a new and very powerful metaprogramming tool, which is a list of types. He deals with implementations of design patterns, such as Visitors, Singleton, Command and Abstract Plant, and the implementation of a multi-viewable mechanism. Combining extraordinary ingenuity with technical virtuosity, it presents an innovative approach to program design. This is the address of the website created by the translator of this book: http://www.nowoczesne-cplusplus.com/ Table of Contents Preface by Scott Meyers Foreword by John Ylissides Admission thanks Part I. Techniques 1. Classes parameterized by guidelines 1.1. The issue of multiplicity in software design 1.2. Failure of universal interfaces 1.3. Wieloddzikenie goes to the rescue? 1.4. Hopes in the templates 1.5. Guidelines and classes of guidelines 1.6. Enhanced guidelines 1.7. Destructors guidelines 1.8. Optional functionality through incomplete specialization 1.9. Connecting guidelines 1.10. Modify the structure of the class using guidelines 1.11. Compatibility of the guidelines 1.12. Class distribution for guidelines 1.13. Summary 2. Techniques 2.1. Static assertions 2.2. Partial specialization of templates 2.3. Local classes 2.4. Mapping of integer constants into types 2.5. Type mapping in type 2.6. Choice of type 2.7. Static detection of inheritance and conversion capabilities 2.8. Packaging for type_info 2.9. NullType and EmptyType 2.10. Characterization of types 2.11. Summary 3. Type lists 3.1. Need 3.2. Defining a list of types 3.3. Creating a list of types by enumerating elements 3.4. Length calculation 3.5. Intermezzo 3.6. Indexing 3.7. Search in type lists 3.8. Combining list types 3.9. Delete an item from the list of types 3.10. Removing repetitions 3.11. Replacing an element in a list of types 3.12. Sorting type lists by partial order 3.13. Generating classes using type lists 3.14. Summary 3.15. Type lists at a glance 4. Assigner of small objects 4.1. The default heap allocator 4.2. Operation of the memory accessory 4.3. Assigner of small objects 4.4. Pieces of memory (chunks) 4.5. Block allocator with fixed size 4.6. SmallObjAllocator class 4.7. We pretend to be the creator of the compiler 4.8. Simple, complicated and yet simple at the end 4.9. Administrative matters 4.10. Summary 4.11. Assigner of small objects at a glance Part II. components 5. Generalized dissepers 5.1. Design pattern. Command 5.2. Command in practice 5.3. Creatives created in C ++ 5.4. Skeleton of the Functor template 5.5. Passing Functor :: operator () 5.6. Operation of functors 5.7. Build one, the other you will receive as a gift 5.8. Conversions of argument and result types 5.9. Indicators for component functions 5.10. Tie 5.11. Macros 5.12. The brutal reality I: the cost of transferring functions 5.13. Brutal reality II: memory allocation on the heap 5.14. Implement "repeat" and "undo" using the Functor template 5.15. Summary 5.16. Functor in a nutshell 6. Implementation of singletons 6.1. Static data + static functions! = Singleton 6.2. Basic C ++ idioms related to singletons 6.3. Ensuring the singleton's uniqueness 6.4. Destroying singleton 6.5. The problem of dead reference 6.6. Solution to the problem of dead references (I): Singleton Feniksowy 6.7. Solution to the problem of dead references (II): Singletons with longevity 6.8. Implementation of singletons with longevity 6.9. multithreading 6.10. We put everything together 6.11. Work with the SingletonHolder template 6.12. Summary 6.13. SingletonHolder in a nutshell 7. Inteligente indicators 7.1. 1001 trinkets about smart indicators 7.2. Profit 7.3. link 7.4. Component functions of intelligent indicators 7.5. Property management strategies 7.6. Operator to download the address 7.7. Implicit conversion to the indicator 7.8. Equality and inequality 7.9. Comparisons 7.10. Control and reporting of errors 7.11. Constant smart indicators and smart pointers to fixed 7.12. Tables 7.13. Intelligent indicators and multithreading 7.14. We put together a generic intelligent indicator 7.15. Summary 7.16. SmartPtr in a nutshell 8. Factories of objects 8.1. Demand for factory facilities 8.2. Factory objects in C ++: classes and objects 8.3. Implementation of the object factory 8.4. Type identifiers 8.5. Generalization 8.6. Szczególiki 8.7. Clone factories 8.8. Using factories of objects in combination with other generic components 8.9. Summary 8.10. The Factory template at a glance 8.11. The CloneFactory template at a glance 9. Abstract Plant 9.1. The role of abstract factories in system architecture 9.2. Generic abstract factory interface 9.3. Implementation of the AbstractFactory interface 9.4. Implementation of an abstract factory based on prototypes 9.5. Summary 9.6. AbstractFactory and ConcreteFactory at a glance 10. Visitors 10.1. The Basics 10.2. Overloading: universal function 10.3. Improved implementation: Acyclic visitors 10.4. Generic implementation of the Visitors pattern 10.5. Return to the "cyclic" version 10.6. Pattern varieties Visitors 10.7. Summary 10.8. Pattern Visitors at a glance 11. Wielometody 11.1. What are multiple dimensions? 11.2. When are the multimeters really needed? 11.3. Force solution: two types of queries 11.4. Automated power solution 11.5. Symmetry in a strength solution 11.6. Logarithmic solution 11.7. FnDispatcher and symmetry 11.8. Funktory as multimodels 11.9. Converting arguments: static_cast or dynamic_cast? 11.10. Fixed solution: speed above all 11.11. BasicDispatcher and BasicFastDispatcher as guidelines 11.12. What's next? 11.13. CONCLUSION 11.14. Wielometody in a nutshell Addition. A minimalist multi-threaded library Dl Criticism of multithreading D.2. The approach used in the Loki library D.3. Atomic operations on integer types D.4. mutexes D.5. Blocking semantics in object-oriented programming D.6. Optional volatile modifier D.7. Semaphores, events and other luxuries D.8. Summary Literature Index |
LPG / Isobutane / Propane Gas Sensor MQ-6
No product available!
No product available!
No product available!
Generic Linear Actuator with Feedback: 12" Stroke, 12V, 1.5"/s
No product available!
Kit for building an intelligent JetBot robot based on Jetson Nano. It consists of Jetson Nano with 2GB RAM, expansion module, WiFi card, camera, motors, housing and a set of mounting screws. JetBot AI Kit (USB WiFi) 2GB
No product available!
No product available!
No product available!
The time has come! For years, we\'ve been hearing the same question again and again from our loyal customers: "Can Ugears create a working clock model kit, one that actually keeps accurate time?" A working mechanical wooden clock has long been our #1 "most-requested" new design. Your dream has also been our dream, and our team of dedicated Ugears engineers and designers has been working hard to bring this shared dream into reality. Finally, the wait is over. Ugears is proud to present the Aero Clock, a fully-functioning DIY wall clock with pendulum. UGears 70154
No product available!
No product available!
No product available!
No product available!
Gravity: UART A6 GSM & GPRS Module is a board with a GSM / GPRS module powered by 5V. It allows sending text messages, making voice calls and transmitting data. DFRobot TEL0113
No product available!
Wired mouse with a precise optical sensor. It has a button to change the resolution in four levels: 800/1200/1600/2400 DPI. 1.8m cable with USB plug. Natec NMY-0667
No product available!
Two-channel DC motor controller for DC motors or one step motor control, built on the L293D circuit with additional ESD protection
No product available!
No product available!
No product available!